Clustering#
Clustering seeks to group data into clusters based on their properties and then allow us to predict which cluster a new member belongs.
import numpy as np
import matplotlib.pyplot as plt
Preparing the data#
We’ll use a dataset generator that is part of scikit-learn called make_moons
. This generates data that falls into 2 different sets with a shape that looks like half-moons.
from sklearn import datasets
def generate_data():
xvec, val = datasets.make_moons(200, noise=0.15)
# encode the output to be 2 elements
x = []
v = []
for xv, vv in zip(xvec, val):
x.append(np.array(xv))
v.append(vv)
return np.array(x), np.array(v)
Tip
By adjusting the noise
parameter, we can blur the boundary between the two datasets, making the classification harder.
x, v = generate_data()
Let’s look at a point and it’s value
print(f"x = {x[0]}, value = {v[0]}")
x = [ 0.61485765 -0.51314619], value = 1
Now let’s plot the data
def plot_data(x, v):
xpt = [q[0] for q in x]
ypt = [q[1] for q in x]
fig, ax = plt.subplots()
ax.scatter(xpt, ypt, s=40, c=v, cmap="viridis")
ax.set_aspect("equal")
return fig
fig = plot_data(x, v)
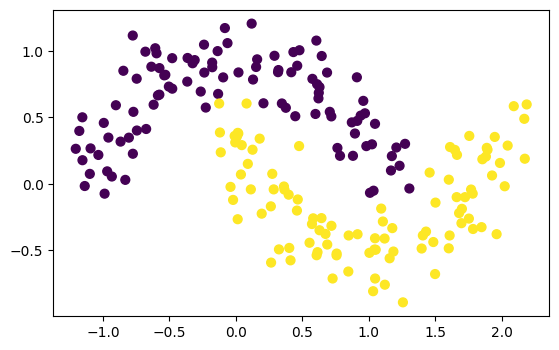
We want to partition this domain into 2 regions, such that when we come in with a new point, we know which group it belongs to.
Constructing the network#
First we setup and train our network
from keras.models import Sequential
from keras.layers import Input, Dense, Dropout, Activation
from keras.optimizers import RMSprop
2025-06-27 16:25:41.060893: I external/local_xla/xla/tsl/cuda/cudart_stub.cc:32] Could not find cuda drivers on your machine, GPU will not be used.
2025-06-27 16:25:41.064093: I external/local_xla/xla/tsl/cuda/cudart_stub.cc:32] Could not find cuda drivers on your machine, GPU will not be used.
2025-06-27 16:25:41.072774: E external/local_xla/xla/stream_executor/cuda/cuda_fft.cc:467] Unable to register cuFFT factory: Attempting to register factory for plugin cuFFT when one has already been registered
WARNING: All log messages before absl::InitializeLog() is called are written to STDERR
E0000 00:00:1751041541.087438 4984 cuda_dnn.cc:8579] Unable to register cuDNN factory: Attempting to register factory for plugin cuDNN when one has already been registered
E0000 00:00:1751041541.091603 4984 cuda_blas.cc:1407] Unable to register cuBLAS factory: Attempting to register factory for plugin cuBLAS when one has already been registered
W0000 00:00:1751041541.103351 4984 computation_placer.cc:177] computation placer already registered. Please check linkage and avoid linking the same target more than once.
W0000 00:00:1751041541.103364 4984 computation_placer.cc:177] computation placer already registered. Please check linkage and avoid linking the same target more than once.
W0000 00:00:1751041541.103366 4984 computation_placer.cc:177] computation placer already registered. Please check linkage and avoid linking the same target more than once.
W0000 00:00:1751041541.103368 4984 computation_placer.cc:177] computation placer already registered. Please check linkage and avoid linking the same target more than once.
2025-06-27 16:25:41.107530: I tensorflow/core/platform/cpu_feature_guard.cc:210] This TensorFlow binary is optimized to use available CPU instructions in performance-critical operations.
To enable the following instructions: AVX2 FMA, in other operations, rebuild TensorFlow with the appropriate compiler flags.
model = Sequential()
model.add(Input((2,)))
model.add(Dense(50, activation="relu"))
model.add(Dense(20, activation="relu"))
model.add(Dense(1, activation="sigmoid"))
2025-06-27 16:25:42.973549: E external/local_xla/xla/stream_executor/cuda/cuda_platform.cc:51] failed call to cuInit: INTERNAL: CUDA error: Failed call to cuInit: UNKNOWN ERROR (303)
rms = RMSprop()
model.compile(loss='binary_crossentropy',
optimizer=rms, metrics=['accuracy'])
model.summary()
Model: "sequential"
┏━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━┳━━━━━━━━━━━━━━━━━━━━━━━━┳━━━━━━━━━━━━━━━┓ ┃ Layer (type) ┃ Output Shape ┃ Param # ┃ ┡━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━╇━━━━━━━━━━━━━━━━━━━━━━━━╇━━━━━━━━━━━━━━━┩ │ dense (Dense) │ (None, 50) │ 150 │ ├─────────────────────────────────┼────────────────────────┼───────────────┤ │ dense_1 (Dense) │ (None, 20) │ 1,020 │ ├─────────────────────────────────┼────────────────────────┼───────────────┤ │ dense_2 (Dense) │ (None, 1) │ 21 │ └─────────────────────────────────┴────────────────────────┴───────────────┘
Total params: 1,191 (4.65 KB)
Trainable params: 1,191 (4.65 KB)
Non-trainable params: 0 (0.00 B)
Training#
Important
We seem to need a lot of epochs here to get a good result
results = model.fit(x, v, batch_size=50, epochs=200, verbose=2)
Epoch 1/200
4/4 - 0s - 110ms/step - accuracy: 0.6150 - loss: 0.6913
Epoch 2/200
4/4 - 0s - 6ms/step - accuracy: 0.7700 - loss: 0.6450
Epoch 3/200
4/4 - 0s - 6ms/step - accuracy: 0.7950 - loss: 0.6172
Epoch 4/200
4/4 - 0s - 10ms/step - accuracy: 0.8300 - loss: 0.5927
Epoch 5/200
4/4 - 0s - 7ms/step - accuracy: 0.8300 - loss: 0.5717
Epoch 6/200
4/4 - 0s - 6ms/step - accuracy: 0.8500 - loss: 0.5525
Epoch 7/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.5343
Epoch 8/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.5172
Epoch 9/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.5011
Epoch 10/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.4868
Epoch 11/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.4728
Epoch 12/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.4608
Epoch 13/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.4490
Epoch 14/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.4377
Epoch 15/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.4281
Epoch 16/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.4181
Epoch 17/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.4096
Epoch 18/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.4010
Epoch 19/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.3933
Epoch 20/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.3854
Epoch 21/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.3788
Epoch 22/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.3722
Epoch 23/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.3659
Epoch 24/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.3596
Epoch 25/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.3540
Epoch 26/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.3488
Epoch 27/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.3431
Epoch 28/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.3385
Epoch 29/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.3333
Epoch 30/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.3293
Epoch 31/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.3246
Epoch 32/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.3203
Epoch 33/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.3164
Epoch 34/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.3128
Epoch 35/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.3090
Epoch 36/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.3059
Epoch 37/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.3016
Epoch 38/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.2986
Epoch 39/200
4/4 - 0s - 6ms/step - accuracy: 0.8700 - loss: 0.2945
Epoch 40/200
4/4 - 0s - 6ms/step - accuracy: 0.8700 - loss: 0.2921
Epoch 41/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.2891
Epoch 42/200
4/4 - 0s - 6ms/step - accuracy: 0.8550 - loss: 0.2865
Epoch 43/200
4/4 - 0s - 6ms/step - accuracy: 0.8700 - loss: 0.2850
Epoch 44/200
4/4 - 0s - 6ms/step - accuracy: 0.8700 - loss: 0.2815
Epoch 45/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.2796
Epoch 46/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2774
Epoch 47/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.2746
Epoch 48/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2725
Epoch 49/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2732
Epoch 50/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2692
Epoch 51/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2665
Epoch 52/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.2649
Epoch 53/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2643
Epoch 54/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2610
Epoch 55/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2607
Epoch 56/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2577
Epoch 57/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2574
Epoch 58/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2543
Epoch 59/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2547
Epoch 60/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2514
Epoch 61/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2526
Epoch 62/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2486
Epoch 63/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2480
Epoch 64/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2477
Epoch 65/200
4/4 - 0s - 7ms/step - accuracy: 0.8600 - loss: 0.2446
Epoch 66/200
4/4 - 0s - 7ms/step - accuracy: 0.8600 - loss: 0.2437
Epoch 67/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2444
Epoch 68/200
4/4 - 0s - 6ms/step - accuracy: 0.8700 - loss: 0.2409
Epoch 69/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2398
Epoch 70/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2387
Epoch 71/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.2385
Epoch 72/200
4/4 - 0s - 7ms/step - accuracy: 0.8600 - loss: 0.2363
Epoch 73/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.2357
Epoch 74/200
4/4 - 0s - 6ms/step - accuracy: 0.8600 - loss: 0.2344
Epoch 75/200
4/4 - 0s - 6ms/step - accuracy: 0.8750 - loss: 0.2342
Epoch 76/200
4/4 - 0s - 6ms/step - accuracy: 0.8650 - loss: 0.2313
Epoch 77/200
4/4 - 0s - 6ms/step - accuracy: 0.8750 - loss: 0.2311
Epoch 78/200
4/4 - 0s - 6ms/step - accuracy: 0.8800 - loss: 0.2303
Epoch 79/200
4/4 - 0s - 6ms/step - accuracy: 0.8750 - loss: 0.2287
Epoch 80/200
4/4 - 0s - 6ms/step - accuracy: 0.8700 - loss: 0.2270
Epoch 81/200
4/4 - 0s - 7ms/step - accuracy: 0.8800 - loss: 0.2257
Epoch 82/200
4/4 - 0s - 7ms/step - accuracy: 0.8850 - loss: 0.2244
Epoch 83/200
4/4 - 0s - 7ms/step - accuracy: 0.8700 - loss: 0.2253
Epoch 84/200
4/4 - 0s - 7ms/step - accuracy: 0.8850 - loss: 0.2220
Epoch 85/200
4/4 - 0s - 7ms/step - accuracy: 0.8850 - loss: 0.2207
Epoch 86/200
4/4 - 0s - 6ms/step - accuracy: 0.8900 - loss: 0.2197
Epoch 87/200
4/4 - 0s - 7ms/step - accuracy: 0.8900 - loss: 0.2181
Epoch 88/200
4/4 - 0s - 7ms/step - accuracy: 0.8900 - loss: 0.2217
Epoch 89/200
4/4 - 0s - 6ms/step - accuracy: 0.8950 - loss: 0.2162
Epoch 90/200
4/4 - 0s - 6ms/step - accuracy: 0.8950 - loss: 0.2150
Epoch 91/200
4/4 - 0s - 6ms/step - accuracy: 0.8950 - loss: 0.2146
Epoch 92/200
4/4 - 0s - 6ms/step - accuracy: 0.8950 - loss: 0.2133
Epoch 93/200
4/4 - 0s - 6ms/step - accuracy: 0.8950 - loss: 0.2137
Epoch 94/200
4/4 - 0s - 6ms/step - accuracy: 0.8900 - loss: 0.2128
Epoch 95/200
4/4 - 0s - 6ms/step - accuracy: 0.8950 - loss: 0.2110
Epoch 96/200
4/4 - 0s - 6ms/step - accuracy: 0.8950 - loss: 0.2096
Epoch 97/200
4/4 - 0s - 6ms/step - accuracy: 0.8950 - loss: 0.2077
Epoch 98/200
4/4 - 0s - 6ms/step - accuracy: 0.8950 - loss: 0.2128
Epoch 99/200
4/4 - 0s - 6ms/step - accuracy: 0.8950 - loss: 0.2076
Epoch 100/200
4/4 - 0s - 6ms/step - accuracy: 0.9000 - loss: 0.2050
Epoch 101/200
4/4 - 0s - 6ms/step - accuracy: 0.9000 - loss: 0.2074
Epoch 102/200
4/4 - 0s - 6ms/step - accuracy: 0.8950 - loss: 0.2041
Epoch 103/200
4/4 - 0s - 6ms/step - accuracy: 0.9050 - loss: 0.2027
Epoch 104/200
4/4 - 0s - 6ms/step - accuracy: 0.9050 - loss: 0.2028
Epoch 105/200
4/4 - 0s - 7ms/step - accuracy: 0.9050 - loss: 0.2016
Epoch 106/200
4/4 - 0s - 7ms/step - accuracy: 0.9100 - loss: 0.1999
Epoch 107/200
4/4 - 0s - 6ms/step - accuracy: 0.9100 - loss: 0.1998
Epoch 108/200
4/4 - 0s - 7ms/step - accuracy: 0.9100 - loss: 0.1975
Epoch 109/200
4/4 - 0s - 7ms/step - accuracy: 0.9100 - loss: 0.1965
Epoch 110/200
4/4 - 0s - 6ms/step - accuracy: 0.9250 - loss: 0.1989
Epoch 111/200
4/4 - 0s - 7ms/step - accuracy: 0.9100 - loss: 0.1953
Epoch 112/200
4/4 - 0s - 7ms/step - accuracy: 0.9200 - loss: 0.1968
Epoch 113/200
4/4 - 0s - 7ms/step - accuracy: 0.9200 - loss: 0.1931
Epoch 114/200
4/4 - 0s - 7ms/step - accuracy: 0.9150 - loss: 0.1928
Epoch 115/200
4/4 - 0s - 7ms/step - accuracy: 0.9200 - loss: 0.1935
Epoch 116/200
4/4 - 0s - 7ms/step - accuracy: 0.9200 - loss: 0.1904
Epoch 117/200
4/4 - 0s - 7ms/step - accuracy: 0.9200 - loss: 0.1886
Epoch 118/200
4/4 - 0s - 7ms/step - accuracy: 0.9200 - loss: 0.1884
Epoch 119/200
4/4 - 0s - 7ms/step - accuracy: 0.9250 - loss: 0.1866
Epoch 120/200
4/4 - 0s - 7ms/step - accuracy: 0.9250 - loss: 0.1861
Epoch 121/200
4/4 - 0s - 7ms/step - accuracy: 0.9250 - loss: 0.1839
Epoch 122/200
4/4 - 0s - 7ms/step - accuracy: 0.9300 - loss: 0.1843
Epoch 123/200
4/4 - 0s - 7ms/step - accuracy: 0.9300 - loss: 0.1813
Epoch 124/200
4/4 - 0s - 7ms/step - accuracy: 0.9400 - loss: 0.1826
Epoch 125/200
4/4 - 0s - 7ms/step - accuracy: 0.9450 - loss: 0.1805
Epoch 126/200
4/4 - 0s - 7ms/step - accuracy: 0.9250 - loss: 0.1775
Epoch 127/200
4/4 - 0s - 7ms/step - accuracy: 0.9450 - loss: 0.1767
Epoch 128/200
4/4 - 0s - 7ms/step - accuracy: 0.9350 - loss: 0.1772
Epoch 129/200
4/4 - 0s - 6ms/step - accuracy: 0.9250 - loss: 0.1778
Epoch 130/200
4/4 - 0s - 6ms/step - accuracy: 0.9450 - loss: 0.1734
Epoch 131/200
4/4 - 0s - 6ms/step - accuracy: 0.9400 - loss: 0.1727
Epoch 132/200
4/4 - 0s - 6ms/step - accuracy: 0.9500 - loss: 0.1725
Epoch 133/200
4/4 - 0s - 7ms/step - accuracy: 0.9450 - loss: 0.1709
Epoch 134/200
4/4 - 0s - 7ms/step - accuracy: 0.9450 - loss: 0.1697
Epoch 135/200
4/4 - 0s - 7ms/step - accuracy: 0.9450 - loss: 0.1679
Epoch 136/200
4/4 - 0s - 7ms/step - accuracy: 0.9400 - loss: 0.1687
Epoch 137/200
4/4 - 0s - 7ms/step - accuracy: 0.9500 - loss: 0.1650
Epoch 138/200
4/4 - 0s - 6ms/step - accuracy: 0.9450 - loss: 0.1655
Epoch 139/200
4/4 - 0s - 6ms/step - accuracy: 0.9450 - loss: 0.1657
Epoch 140/200
4/4 - 0s - 7ms/step - accuracy: 0.9500 - loss: 0.1626
Epoch 141/200
4/4 - 0s - 7ms/step - accuracy: 0.9500 - loss: 0.1612
Epoch 142/200
4/4 - 0s - 7ms/step - accuracy: 0.9550 - loss: 0.1592
Epoch 143/200
4/4 - 0s - 6ms/step - accuracy: 0.9500 - loss: 0.1589
Epoch 144/200
4/4 - 0s - 6ms/step - accuracy: 0.9450 - loss: 0.1575
Epoch 145/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1575
Epoch 146/200
4/4 - 0s - 6ms/step - accuracy: 0.9450 - loss: 0.1562
Epoch 147/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1535
Epoch 148/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1514
Epoch 149/200
4/4 - 0s - 6ms/step - accuracy: 0.9500 - loss: 0.1546
Epoch 150/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1495
Epoch 151/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1495
Epoch 152/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1492
Epoch 153/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1462
Epoch 154/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1450
Epoch 155/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1435
Epoch 156/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1433
Epoch 157/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1411
Epoch 158/200
4/4 - 0s - 7ms/step - accuracy: 0.9600 - loss: 0.1408
Epoch 159/200
4/4 - 0s - 7ms/step - accuracy: 0.9550 - loss: 0.1396
Epoch 160/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1376
Epoch 161/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1357
Epoch 162/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1344
Epoch 163/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1326
Epoch 164/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1327
Epoch 165/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1311
Epoch 166/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1312
Epoch 167/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1279
Epoch 168/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1266
Epoch 169/200
4/4 - 0s - 6ms/step - accuracy: 0.9550 - loss: 0.1287
Epoch 170/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1239
Epoch 171/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1244
Epoch 172/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1217
Epoch 173/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1214
Epoch 174/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1208
Epoch 175/200
4/4 - 0s - 7ms/step - accuracy: 0.9600 - loss: 0.1193
Epoch 176/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1184
Epoch 177/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1168
Epoch 178/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1168
Epoch 179/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1148
Epoch 180/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1142
Epoch 181/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1145
Epoch 182/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1110
Epoch 183/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1119
Epoch 184/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1100
Epoch 185/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1078
Epoch 186/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1085
Epoch 187/200
4/4 - 0s - 7ms/step - accuracy: 0.9600 - loss: 0.1056
Epoch 188/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1050
Epoch 189/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1048
Epoch 190/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1026
Epoch 191/200
4/4 - 0s - 6ms/step - accuracy: 0.9650 - loss: 0.1028
Epoch 192/200
4/4 - 0s - 6ms/step - accuracy: 0.9650 - loss: 0.1030
Epoch 193/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.1006
Epoch 194/200
4/4 - 0s - 6ms/step - accuracy: 0.9650 - loss: 0.1008
Epoch 195/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.0984
Epoch 196/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.0975
Epoch 197/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.0971
Epoch 198/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.0952
Epoch 199/200
4/4 - 0s - 6ms/step - accuracy: 0.9650 - loss: 0.0960
Epoch 200/200
4/4 - 0s - 6ms/step - accuracy: 0.9600 - loss: 0.0944
score = model.evaluate(x, v, verbose=0)
print(f"score = {score[0]}")
print(f"accuracy = {score[1]}")
score = 0.09192071110010147
accuracy = 0.9700000286102295
Predicting#
Let’s look at a prediction. We need to feed in a single point as an array of shape (N, 2)
, where N
is the number of points
res = model.predict(np.array([[-2, 2]]))
res
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 32ms/step
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 42ms/step
array([[1.2150591e-17]], dtype=float32)
We see that we get a floating point number. We will need to convert this to 0 or 1 by rounding.
Let’s plot the partitioning
M = 256
N = 256
xmin = -1.75
xmax = 2.5
ymin = -1.25
ymax = 1.75
xpt = np.linspace(xmin, xmax, M)
ypt = np.linspace(ymin, ymax, N)
To make the prediction go faster, we want to feed in a vector of these points, of the form:
[[xpt[0], ypt[0]],
[xpt[1], ypt[1]],
...
]
We can see that this packs them into the vector
pairs = np.array(np.meshgrid(xpt, ypt)).T.reshape(-1, 2)
pairs[0]
array([-1.75, -1.25])
Now we do the prediction. We will get a vector out, which we reshape to match the original domain.
res = model.predict(pairs, verbose=0)
res.shape = (M, N)
Finally, round to 0 or 1
domain = np.where(res > 0.5, 1, 0)
and we can plot the data
fig, ax = plt.subplots()
ax.imshow(domain.T, origin="lower",
extent=[xmin, xmax, ymin, ymax], alpha=0.25)
xpt = [q[0] for q in x]
ypt = [q[1] for q in x]
ax.scatter(xpt, ypt, s=40, c=v, cmap="viridis")
<matplotlib.collections.PathCollection at 0x7f53d8e85a50>
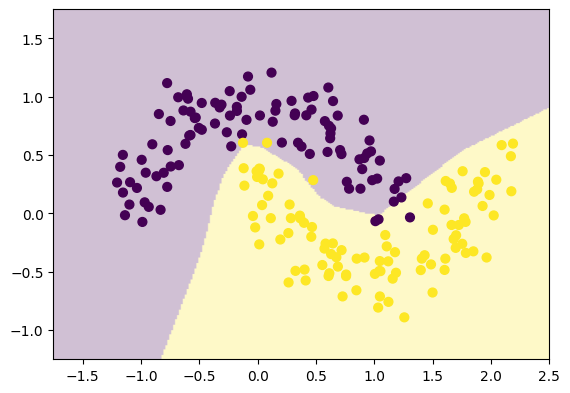