Application: Estimating \(H_0\) from Type Ia Supernovae#
Type Ia supernova are used as standardizable candles to measure cosmological distances. By observing the lightcurve and measuring how long it takes for the supernova to dim we can empirically determine its brightness via the Phillips relation.
The paper Spectra and Hubble Space Telescope Light Curves of Six Type Ia Supernovae at 0.511 < z < 1.12 and the Union2 Compilation by Amanullah et al. made a data set available that has ~ 500 Type Ia supernovae.
Note
That paper does a far, far more sophisticated analysis than we do, and they fit for other cosmological parameters that we will, so we will not get the same answer as they do. But this is a fun dataset to try out regression with.
import numpy as np
import matplotlib.pyplot as plt
The dataset has 4 columns:
supernova identifier
redshift, \(z\), due to cosmological expansion
distance modulus, \(\mu\), defined as:
\[\mu = m - M = 5 \log_{10} \left (\frac{d}{10~\mbox{pc}}\right )\]where \(m\) is the apparent magnitude of the supernova (what we observe) and \(M\) is the absolute magnitiude of the supernova (inferred empirically from the lightcurve).
uncertainty in \(\mu\)
data = np.genfromtxt("SCPUnion2_mu_vs_z.txt",
dtype=[("name", "S6"), ("z", "f8"), ("mu", "f8"), ("dmu", "f8")])
let’s sort the data based on z
idx_sort = np.argsort(data["z"])
zs = data["z"][idx_sort]
mus = data["mu"][idx_sort]
dmus = data["dmu"][idx_sort]
Now let’s plot the distance modulus vs redshift
fig, ax = plt.subplots()
ax.errorbar(data["z"], data["mu"], yerr=data["dmu"], fmt="o", ms=4)
ax.set_xlabel("z")
ax.set_ylabel(r"$\mu$")
ax.grid()
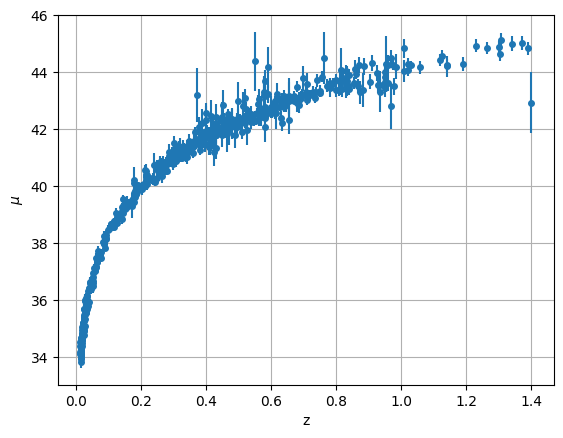
This looks like the top curve of Figure 9.
We can also make a plot that looks a bit more like a Hubble diagram by plotting redshift vs. distance, computing distance as:
fig, ax = plt.subplots()
ax.plot(10 * 10**(data["mu"]/5) / 1.e9, data["z"], "o", ms=4)
ax.set_xlabel("distance (Gpc)")
ax.set_ylabel("z")
ax.grid()
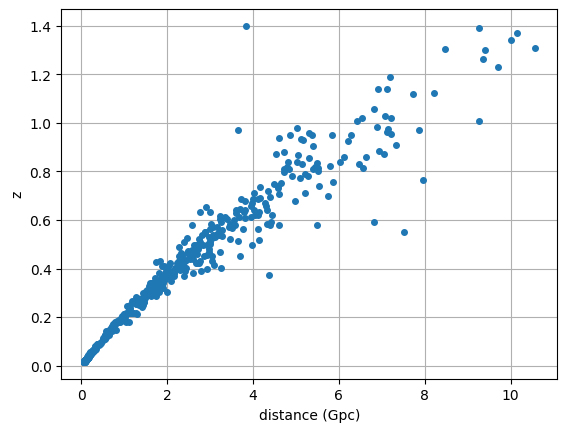
This shows a linear relation between distance and redshift at low redshift.
Cosmological parameters#
We have the distance modulus, which is related to the magnitudes via:
Since cosmologists usually work in terms of Mpc, let’s rewrite this as:
Now, in an expanding Universe, the distance that goes here is the luminosity distance which can be expressed via an expansion in redshift as (for \(z \ll 1\)):
Here \(H_0\) is the Hubble constant and \(q_0\) is the deceleration parameter.
We’ll try to estimate \(H_0\) from this data.
Note
This is different than what the original paper did—they used a value of \(H_0\) to find a \(\Omega_m\) and \(\Omega_\Lambda\) using the more general expression for luminosity distance given in Perlmutter et al. 1997 (see footnote 14):
with \(S(x)\) and \(\kappa\) defined as:
\(\Omega_M + \Omega_\Lambda > 1\): \(S(x) = \sin(x)\); \(\kappa = 1 - \Omega_M - \Omega_\Lambda\)
\(\Omega_M + \Omega_\Lambda = 1\): \(S(x) = x\); \(\kappa = 1\)
\(\Omega_M + \Omega_\Lambda < 1\): \(S(x) = \sinh(x)\); \(\kappa = 1 - \Omega_M - \Omega_\Lambda\)
Using this definition would require us to combine integration and fitting.
Our fit#
We want to fit:
which we’ll write as:
This is a nonlinear expression in terms of the fit parameters, \(a_0\), \(a_1\). Once we get \(a_0\), we can get Hubble’s constant as:
First we need to select only the low redshift data, where our expansion of distance luminosity might apply. We’ll consider \(z < 0.2\).
zmax = 0.2
idx = zs < zmax
z_low = zs[idx]
mu_low = mus[idx]
dmu_low = dmus[idx]
fig, ax = plt.subplots()
ax.errorbar(z_low, mu_low, yerr=dmu_low, fmt="o")
ax.set_xlabel("z")
ax.set_ylabel(r"$\mu$")
ax.grid()
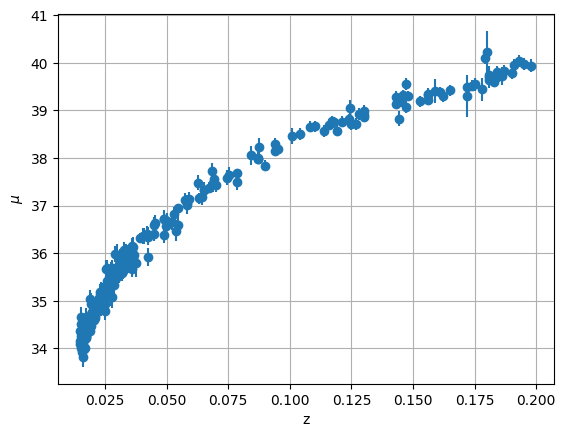
We’ll use the SciPy fitting routine for this. Let’s start by writing the residual that the fit will use.
from scipy import optimize
def resid(avec, z, mu, dmu):
return (mu - (5 * np.log10(avec[0] * z * (1 + 0.5 * (1 - avec[1]) * z)) + 25)) / dmu
Now let’s start with some initial guesses
# imagine H0 = 50
c = 3.e5 # km/s
H0_guess = 50 # km/s/Mpc
a0 = c / H0_guess
a1 = 1
afit, flag = optimize.leastsq(resid, [a0, a1], args=(z_low, mu_low, dmu_low))
afit
array([ 4.30725463e+03, -3.38755447e-01])
From this fit, we can recover the Hubble constant:
H0 = c / afit[0]
H0
np.float64(69.64993387276675)
Finally, let’s plot our fit
mu_fit = 5 * np.log10(afit[0] * z_low * (1 + 0.5 * (1 - afit[1]) * z_low)) + 25
ax.plot(z_low, mu_fit, zorder=100)
fig
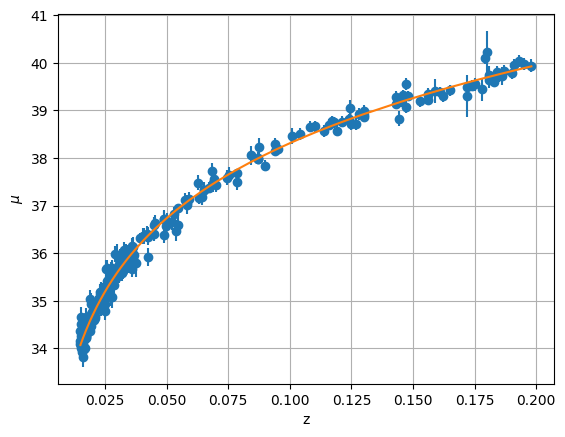
We find a reasonable value of \(H_0 = 69.6~\mathrm{km/s/Mpc}\).