Simple supernova lightcurve#
import numpy as np
import matplotlib.pyplot as plt
A simple model of a supernova lightcurve is just to add up the energy of the photons that result from the decay:
The evolution equations for the number densities appear as:
where \(\lambda_\mathrm{Ni}\) is the decay constant for \({}^{56}\mathrm{Ni}\) and \(\lambda_\mathrm{Co}\) is the decay constant for \({}^{56}\mathrm{Co}\).
The initial conditions are:
We can integrate the Ni equation directly, giving:
then the Co equation becomes:
This can be integrated by applying an integrating factor:
Now the term in the \([ \ldots ]\) is just \(\lambda_\mathrm{Ni} n_\mathrm{Ni}\), so this becomes:
This gives:
With these number densities, we can get the total amount of Ni or Co at any point in time:
and
This gives:
where \(m_{56}\) is just \(56 m_u\), with \(m_u\) the atomic mass unit (this approximates the mass of Ni and Co).
Implementation#
some physical constants
seconds_per_day = 24*3600
erg_per_MeV = 1.6e-12*1.e6
m_u = 1.66e-24 # CGS
solar_mass = 2.e33 # CGS
solar_luminosity = 4.e33 # CGS
the core LightCurve
class
class LightCurve:
def __init__(self, M_exp, *, X_Ni_0=1.0):
"""create the lightcurve. Here, M_exp is the mass of ejecta in grams"""
self.M_exp = M_exp
self.X_Ni_0 = X_Ni_0
# note: see Nadyozhin 1994, ApJ, 92, 527 for Q values of
# just photons. They suggest Q_Ni = 1.75 MeV, Q_Co = 3.73 MeV
thalf_Ni = 6.1 * seconds_per_day # half-life of Ni-56
self.lambda_Ni = np.log(2) / thalf_Ni
self.Q_Ni = 1.75 * erg_per_MeV
self.m_Ni = 56 * m_u
thalf_Co = 77.1*seconds_per_day # half-life of Co-56
self.lambda_Co = np.log(2) / thalf_Co
self.Q_Co = 3.73 * erg_per_MeV
self.m_Co = 56 * m_u
def X_Ni(self, t):
return self.X_Ni_0 * np.exp(-self.lambda_Ni * t)
def X_Co(self, t):
return (self.lambda_Ni / (self.lambda_Co - self.lambda_Ni) * self.X_Ni_0 *
(np.exp(-self.lambda_Ni * t) - np.exp(-self.lambda_Co * t)))
def L(self, t):
return (self.Q_Ni * self.M_exp / self.m_Ni * self.lambda_Ni * self.X_Ni(t) +
self.Q_Co * self.M_exp / self.m_Co * self.lambda_Co * self.X_Co(t))
Lightcurve from \(1~M_\odot\) of \({}^{56}\mathrm{Ni}\)#
# initial condition
M_exp = 1.0*solar_mass
lc = LightCurve(M_exp)
t = np.logspace(np.log10(1.0), np.log10(3.e7), 100)
fig, ax = plt.subplots()
ax.plot(t / seconds_per_day, lc.L(t) / solar_luminosity)
ax.set_xlabel("days")
ax.set_ylabel(r"$L/L_\odot$")
ax.set_yscale("log")
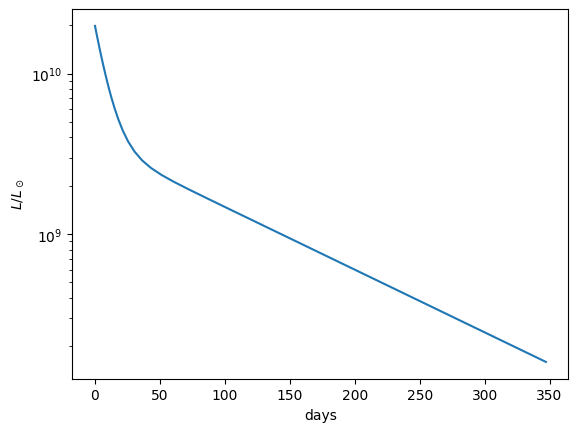
Different masses of \({}^{56}\mathrm{Ni}\)#
fig, ax = plt.subplots()
t = np.logspace(np.log10(1.0), np.log10(3.e7), 100)
for mass in [0.4, 0.6, 0.8, 1.0, 1.2]:
lc = LightCurve(mass * solar_mass)
ax.plot(t / seconds_per_day, lc.L(t) / solar_luminosity,
label=f"$M_\mathrm{{Ni}} = {mass} M_\odot$")
ax.set_yscale("log")
ax.set_xlabel("days")
ax.set_ylabel(r"$L/L_\odot$")
ax.legend()
<matplotlib.legend.Legend at 0x7f3fd01bdc10>
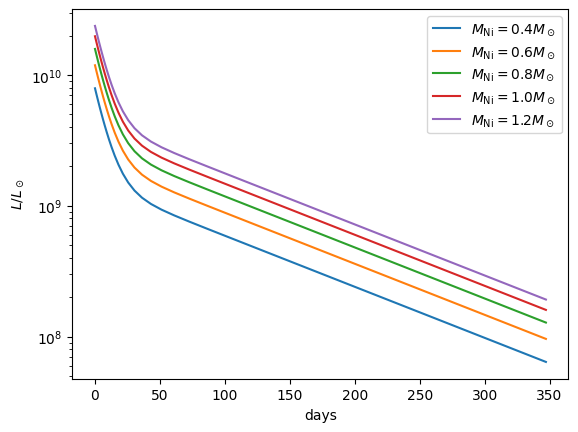